Overview
My team was tasked with enhancing a basic command line interface (CLI) AddressBook for our Software Engineering project.
We decided to morph it into a school module tracker called GradTrak.
GradTrak is an application designed for students of National University of Singapore (NUS) to easily plan and track modules as well as check them against the graduation requirements.
The user interacts with GradTrak mainly through the CLI, but it also has a Graphical User Interface (GUI) created with JavaFX. It is written in Java and has more than ten thousand lines of code.
My role was to design and write the codes for the module recommendation feature, and to enhance the existing module finding feature. The following sections illustrate these contributions in more detail, as well as the relevant sections I have added to the User Guide and Developer Guide.
Summary of contributions
This section summarises my coding and other helpful contributions to GradTrak.
-
Major enhancement: added a module recommendation feature
-
What it does: This feature displays a list of modules which the user is recommended to read based on existing GradTrak modules and graduation requirements specific to the user’s course of study. The list is sorted primarily by the priority of the requirement type satisfied by the recommended module, and secondarily by the level of the module.
-
Justification: This feature significantly benefits users who are unfamiliar with the exact graduation requirements of their course, or are unsure of what modules to read for future semesters. They can simply pick a high-priority module near the top of the recommendation list — this module already has its prerequisites satisfied, contributes to their graduation requirements and will likely serve as a prerequisite for higher level modules. This allows users to plan their modules systematically so that they are on the right track towards graduation.
-
Highlights: The implementation of this feature was challenging as it requires interactions with many components of GradTrak. It needs to retrieve information of all NUS modules, check each module for its preclusions or prerequisites in the user’s module list, and determine whether it contributes to the user’s course requirements and if so, the requirement type of highest priority that it satisfies. In particular, it was necessary to liaise with the implementation of the graduation-checking feature to ensure that the recommended modules actually bring the user closer to graduation.
-
-
Minor enhancement: added additional parameters for the module finding feature, allowing the user to search GradTrak modules by module code, semester, grade or finished status.
-
Code contributed: [Project Code Dashboard]
-
Other contributions:
-
Project management:
-
Documentation:
-
Helped to proofread and polish the language of the User Guide and Developer Guide
-
Edited some old diagrams from AddressBook for the purpose of GradTrak (#80)
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Recommend module: rec
Recommends modules that can be read based on the current module plan and course requirements.
Format: rec
If any change is made to the course or module plan, enter |
The figure below shows a sample recommendation list for a student studying Computer Science Algorithms with an empty module plan.
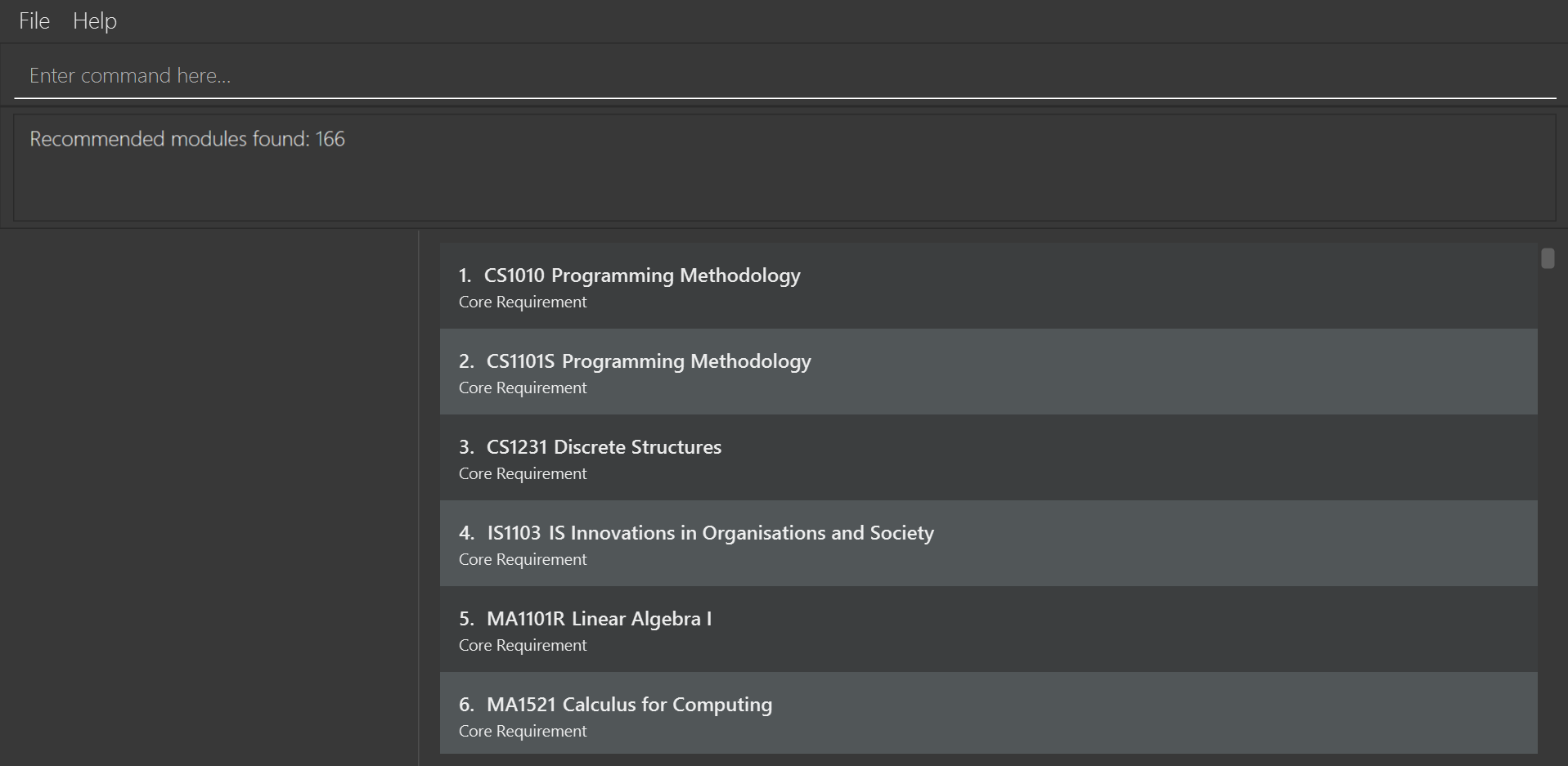
Find module: find
Finds modules in the module plan matching all given module code, semester, grade or finished status.
Format: find [c/MODULE_CODE] [s/SEMESTER] [g/GRADE] [f/IS_FINISHED]
Examples:
-
find c/CS
Lists all modules with "CS" in their codes.
.png)
-
find s/Y1S2
Lists all modules in Y1S2.
.png)
-
find g/A f/y
Lists all finished modules with grade A.
.png)
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Module recommendation feature
The module recommendation feature displays modules which the student is recommended to read based on the current module plan
and specific course requirements. It generates a list of module codes together with their corresponding titles and requirement
types satisfied. The entire list is displayed on the Result Panel upon entering the rec
command.
Modules which satisfy only Unrestricted Electives are not included to prevent the list from being too long. |
Current implementation
Each recommended module is represented by a RecModule
which contains a unique ModuleInfo
and its corresponding
CourseReqType
satisfied, as shown in the diagram below.
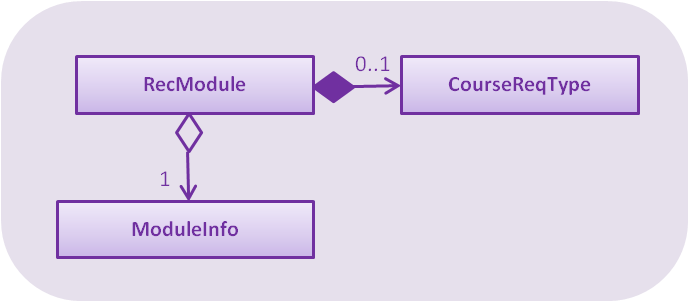
RecModule
class diagramWhen ModelManager
is initialised, Model#getObservableRecModuleList
is called which generates an
ObservableList
of RecModule
, one for each module in the entire ModuleInfoList
. This list is wrapped in a FilteredList
,
which is further wrapped in a SortedList
, both stored in ModelManager
. At this point, all RecModule
in the list contain an empty CourseReqType
field.
When the rec
command is entered, the sequence of execution is as follows:
-
Model#updateRecModuleList
is called, which creates aRecModulePredicate
given the student’sCourse
andReadOnlyGradTrak
, and aRecModuleComparator
. -
The
RecModulePredicate
is applied to theFilteredList
ofRecModule
. In each test:-
An
EligibleModulePredicate
which takes inReadOnlyGradTrak
tests if theModuleInfo
of thisRecModule
is eligible to be read. If the module is already present in the module plan or does not have its prerequisites satisfied, thisRecModule
is filtered out. -
The
ModuleInfoCode
(call itcodeToTest
) of theRecModule
is retrieved.
AnonFailedCodeList
ofModuleInfoCode
corresponding to non-failedModuleTaken
(already passed or to be read in a future semester) is also retrieved fromReadOnlyGradTrak
. -
The
codeToTest
is then passed intoCourse#getCourseReqTypeOf
, which in turn callsCourseRequirement#canFulfill
for eachCourseRequirement
listed inCourse
. A list ofCourseReqType
that thecodeToTest
can satisfy is returned. ThiscourseReqTypeList
is sorted by the priority ofCourseReqType
as defined in theenum
class:CORE
,BD
,IE
,FAC
,GE
. -
For each
CourseReqType
in thecourseReqTypeList
(highest priority first):-
Course#isCodeContributing
is called, which takes in theCourseReqType
,nonFailedCodeList
andcodeToTest
. -
For each
CourseRequirement
listed inCourse
corresponding to the givenCourseReqType
,CourseRequirement#getUnfulfilled
is called which takes in thenonFailedCodeList
and returns anunfulfilledRegexList
of RegExes not satisfied. -
If the
codeToTest
matches any of the RegExes in theunfulfilledRegexList
,Course#isCodeContributing
returnstrue
and the loop forcourseReqTypeList
terminates.
-
-
The
CourseReqType
of highest priority satisfied bycodeToTest
is then set into theRecModule
. However, if thecodeToTest
does not contribute to any of theCourseRequirement
listed inCourse
, thisRecModule
is filtered out.
-
-
The
RecModuleComparator
is applied to theSortedList
ofRecModule
. It sorts the list in decreasing priority of theCourseReqType
satisfied by theRecModule
. ThoseRecModule
with equal priority are sorted by module level (the first numerical digit of itsModuleInfoCode
), considering that lower level modules are usually read first. In the case of equal priority and module level, lexicographical sorting of itsModuleInfoCode
is used. -
The
SortedList
ofRecModule
is retrieved fromModelManager
and displayed to the student in the Result Panel.
If there are changes to ReadOnlyGradTrak
(adding, editing or deleting modules) or Course
(changing the course of study), the rec
command
must be run again to reflect the updated recommendation list.
The sequence diagrams summarising the above execution are shown below.
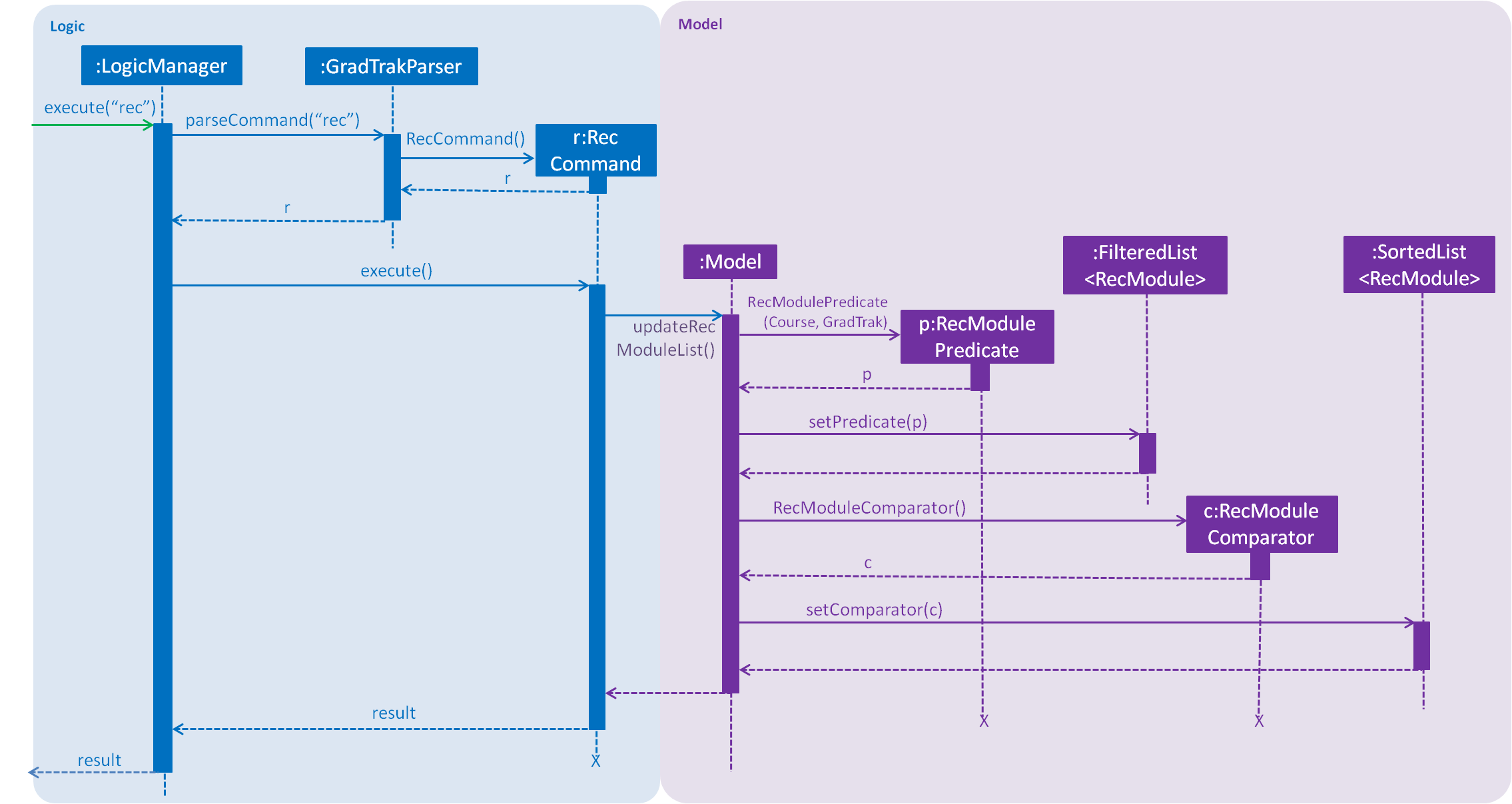
RecCommand
sequence diagram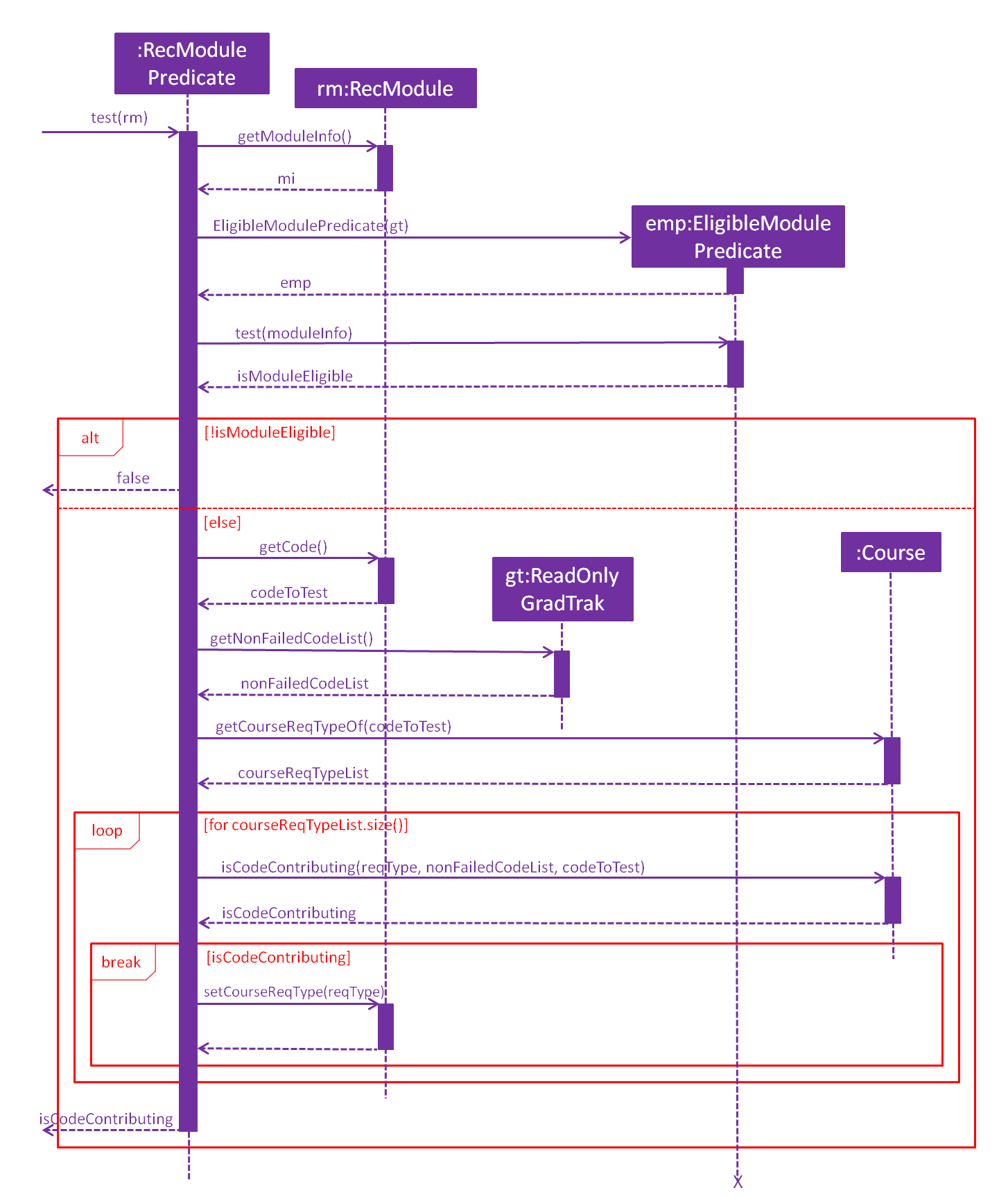
RecModulePredicate
sequence diagram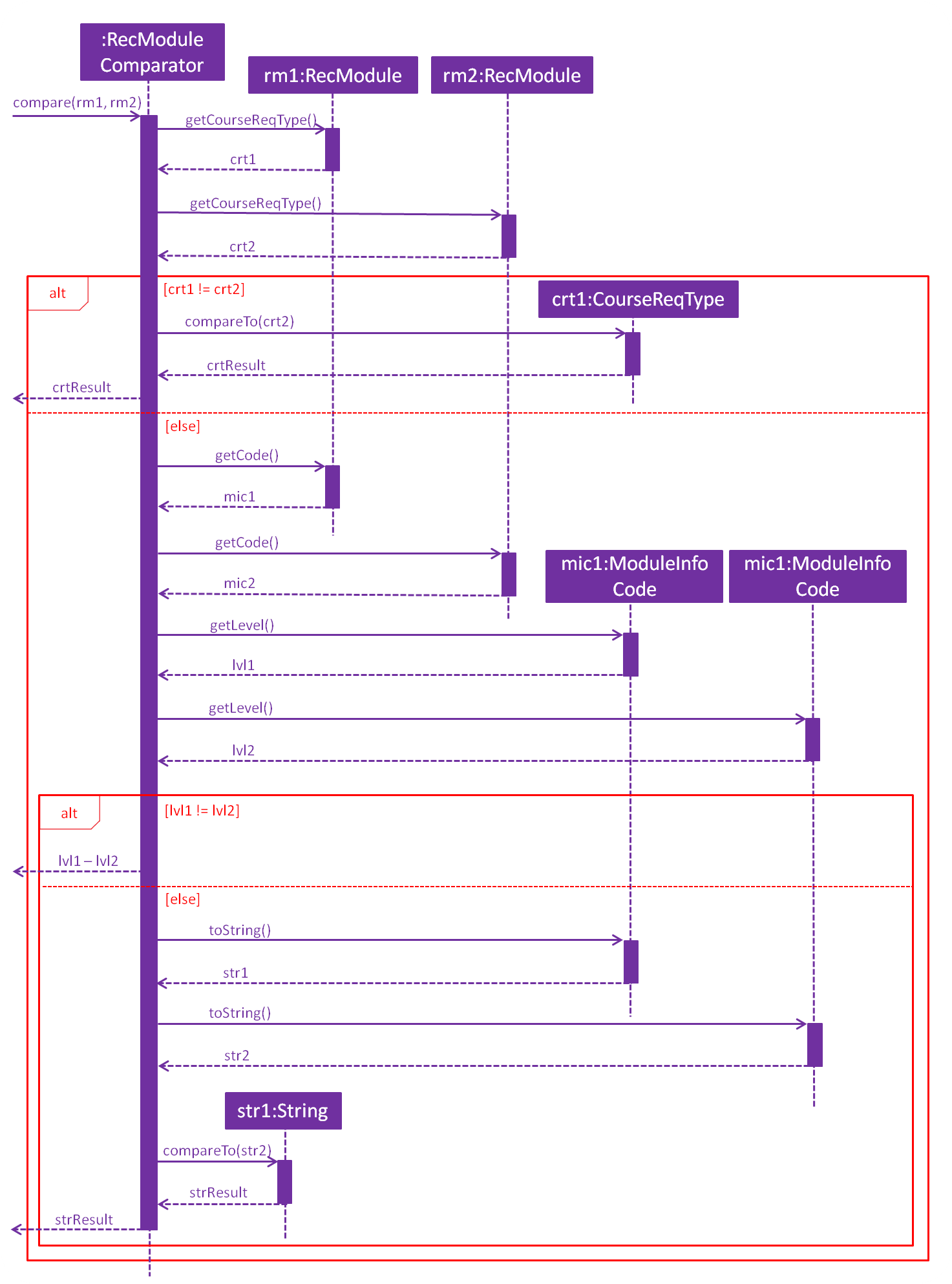
RecModuleComparator
sequence diagramDesign Considerations
Aspect: Sorting of recommendation list
-
Alternative 1 (current choice): Recommendation list is sorted by a fixed order of
CourseReqType
priority as defined in theenum
class-
Pros: Easy to implement and modify
-
Cons: Student may have his own order of priority that differs from the default one
-
-
Alternative 2: Recommendation list can be sorted by a custom order defined by the student
-
Pros: Student can sort the list according to his own preferences
-
Cons: Difficult to implement if several parameters for sorting is allowed; input method for the custom order is problematic
-
Aspect: Format of recommendation
-
Alternative 1 (current choice): Display a list of all eligible modules that contribute to course requirements
-
Pros: Student has a greater freedom of choice
-
Cons: Student may be confused or unable to decide if the list is too long
-
-
Alternative 2: Display
n
modules for each semester, wheren
is decided by the student-
Pros: Student can plan modules for specific semesters easily and quickly
-
Cons: Algorithm required to plan for all semesters can be complex; student may not prefer the given plan
-
Possible Improvements
-
Allow the student to display a module’s information (from
displaymod
command) using its index in the recommendation list -
Allow the student to add a module to the module plan using its index in the recommendation list
-
Enable recommendation of Unrestricted Electives based on personal interests of the user